1) Create FirstScreen .xml
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context=".FirstScreen"
android:orientation="vertical">
<TextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_centerHorizontal="true"
android:layout_centerVertical="true"
android:textColor="#898989"
android:textStyle="bold"
android:textSize="16sp"
android:text="Shopping Cart" />
<LinearLayout
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:orientation="vertical"
android:id="@+id/linearMain">
</LinearLayout>
<Button android:id="@+id/second"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Checkout"/>
</LinearLayout>
2 ) Create Secondscreen.xml
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="vertical" >
<TextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:textColor="#898989"
android:textStyle="bold"
android:textSize="16sp"
android:text="Shopping Cart Content" />
<TextView
android:id="@+id/showCart"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_centerHorizontal="true"
android:layout_centerVertical="true"/>
<Button android:id="@+id/third"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Payment"/>
</LinearLayout>
3 ) Create thirdscrren.xml
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="vertical" >
<TextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:textColor="#898989"
android:textStyle="bold"
android:textSize="16sp"
android:text="Thanks you , you have purchased these products" />
<TextView
android:id="@+id/showCart"
android:layout_width="wrap_content"
android:layout_height="wrap_content"/>
</LinearLayout>
4) Create String.xml
<< res >> values >> string.xml >>
<?xml version="1.0" encoding="utf-8"?>
<resources>
<string name="app_name">MVC Pattern Basics</string>
<string name="menu_settings">Settings</string>
<string name="hello_world">Hello world!</string>
</resources>
5 ) Create Firstscreen.java
package com.androidexample.mvc;
import android.os.Bundle;
import android.util.Log;
import android.view.View;
import android.view.View.OnClickListener;
import android.widget.Button;
import android.widget.LinearLayout;
import android.widget.TextView;
import android.widget.Toast;
import android.app.ActionBar.LayoutParams;
import android.app.Activity;
import android.content.Intent;
public class FirstScreen extends Activity {
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.firstscreen);
final LinearLayout lm = (LinearLayout) findViewById(R.id.linearMain);
final Button secondBtn = (Button) findViewById(R.id.second);
final Controller aController = (Controller) getApplicationContext();
ModelProducts productObject = null;
for(int i=1;i<=4;i++)
{
int price = 10+i;
productObject = new ModelProducts("Product "+i,"Description "+i,price);
aController.setProducts(productObject);}
int ProductsSize = aController.getProductsArraylistSize();
LinearLayout.LayoutParams params = new LinearLayout.LayoutParams(
LayoutParams.WRAP_CONTENT, LayoutParams.WRAP_CONTENT);
for(int j=0;j< ProductsSize;j++)
{
String pName = aController.getProducts(j).getProductName();
int pPrice = aController.getProducts(j).getProductPrice();
LinearLayout ll = new LinearLayout(this);
ll.setOrientation(LinearLayout.HORIZONTAL);
TextView product = new TextView(this);
product.setText(" "+pName+" ");
ll.addView(product);
TextView price = new TextView(this);
price.setText(" $"+pPrice+" ");
ll.addView(price);
final Button btn = new Button(this);
btn.setId(j+1);
btn.setText("Add To Cart");
btn.setLayoutParams(params);
final int index = j;
btn.setOnClickListener(new OnClickListener() {
public void onClick(View v) {
Log.i("TAG", "index :" + index);
ModelProducts tempProductObject = aController.getProducts(index);
if(!aController.getCart().checkProductInCart(tempProductObject))
{
btn.setText("Added");
aController.getCart().setProducts(tempProductObject);
Toast.makeText(getApplicationContext(), "Now Cart size: "+aController.getCart().getCartSize(),
Toast.LENGTH_LONG).show();
}
else
{
Toast.makeText(getApplicationContext(), "Product "+(index+1)+" already added in cart.", Toast.LENGTH_LONG).show();
}
}
});
ll.addView(btn);
lm.addView(ll);
}
secondBtn.setOnClickListener(new OnClickListener() {
public void onClick(View v) {
Intent i = new Intent(getBaseContext(), SecondScreen.class);
startActivity(i);
}
});
}
}
6 ) Create Secondscreen.java
package com.androidexample.mvc;
import android.app.Activity;
import android.content.Intent;
import android.os.Bundle;
import android.view.View;
import android.view.View.OnClickListener;
import android.widget.Button;
import android.widget.TextView;
import android.widget.Toast;
public class SecondScreen extends Activity {
@Override
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.secondscreen);
TextView showCartContent = (TextView) findViewById(R.id.showCart);
final Button thirdBtn = (Button) findViewById(R.id.third);
final Controller aController = (Controller) getApplicationContext();
final int cartSize = aController.getCart().getCartSize();
String showString = "";
if(cartSize >0)
{
for(int i=0;i<cartSize;i++)
{
String pName = aController.getCart().getProducts(i).getProductName();
int pPrice = aController.getCart().getProducts(i).getProductPrice();
String pDisc = aController.getCart().getProducts(i).getProductDesc();
showString += "\n\nProduct Name : "+pName+"\n"+
"Price : "+pPrice+"\n"+
"Discription : "+pDisc+""+
"\n -----------------------------------";
}
}
else
showString = "\n\nShopping cart is empty.\n\n";
showCartContent.setText(showString);
thirdBtn.setOnClickListener(new OnClickListener() {
public void onClick(View v) {
if(cartSize >0)
{
Intent i = new Intent(getBaseContext(), ThirdScreen.class);
startActivity(i);
}
else
Toast.makeText(getApplicationContext(),
"Shopping cart is empty.",
Toast.LENGTH_LONG).show();
}
});
}
@Override
protected void onDestroy() {
super.onDestroy();
}
}
7 ) Create Thirdscreen.java
package com.androidexample.mvc;
import android.app.Activity;
import android.os.Bundle;
import android.widget.TextView;
public class ThirdScreen extends Activity {
@Override
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.thirdscreen);
TextView showCartContent = (TextView) findViewById(R.id.showCart);
final Controller aController = (Controller) getApplicationContext();
int cartSize = aController.getCart().getCartSize();
String showString = "";
for(int i=0;i<cartSize;i++)
{
String pName = aController.getCart().getProducts(i).getProductName();
int pPrice = aController.getCart().getProducts(i).getProductPrice();
String pDisc = aController.getCart().getProducts(i).getProductDesc();
showString += "\n\nProduct Name : "+pName+"\n"+
"Price : "+pPrice+"\n"+
"Discription : "+pDisc+""+
"\n -----------------------------------";
}
showCartContent.setText(showString);
}
}
8) Create Controller.java
package com.androidexample.mvc;
import java.util.ArrayList;
import android.app.Application;
public class Controller extends Application{
private ArrayList<ModelProducts> myProducts = new ArrayList<ModelProducts>();
private ModelCart myCart = new ModelCart();
public ModelProducts getProducts(int pPosition) {
return myProducts.get(pPosition);
}
public void setProducts(ModelProducts Products) {
myProducts.add(Products);
}
public ModelCart getCart() {
return myCart;
}
public int getProductsArraylistSize() {
return myProducts.size();
}
}
9 ) Modelcart.java
package com.androidexample.mvc;
import java.util.ArrayList;
public class ModelCart{
private ArrayList<ModelProducts> cartProducts = new ArrayList<ModelProducts>();
public ModelProducts getProducts(int pPosition) {
return cartProducts.get(pPosition);
}
public void setProducts(ModelProducts Products) {
cartProducts.add(Products);
}
public int getCartSize() {
return cartProducts.size();
}
public boolean checkProductInCart(ModelProducts aProduct) {
return cartProducts.contains(aProduct);
}
}
10 ) Create Modelproducts.java
package com.androidexample.mvc;
public class ModelProducts {
private String productName;
private String productDesc;
private int productPrice;
public ModelProducts(String productName,String productDesc,int productPrice)
{
this.productName = productName;
this.productDesc = productDesc;
this.productPrice = productPrice;
}
public String getProductName() {
return productName;
}
public String getProductDesc() {
return productDesc;
}
public int getProductPrice() {
return productPrice;
}
}
output with Screenshots:
online cart 1;
online cart 2:
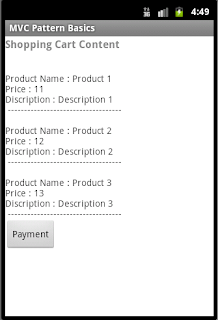
online cart 3:
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context=".FirstScreen"
android:orientation="vertical">
<TextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_centerHorizontal="true"
android:layout_centerVertical="true"
android:textColor="#898989"
android:textStyle="bold"
android:textSize="16sp"
android:text="Shopping Cart" />
<LinearLayout
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:orientation="vertical"
android:id="@+id/linearMain">
</LinearLayout>
<Button android:id="@+id/second"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Checkout"/>
</LinearLayout>
2 ) Create Secondscreen.xml
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="vertical" >
<TextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:textColor="#898989"
android:textStyle="bold"
android:textSize="16sp"
android:text="Shopping Cart Content" />
<TextView
android:id="@+id/showCart"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_centerHorizontal="true"
android:layout_centerVertical="true"/>
<Button android:id="@+id/third"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Payment"/>
</LinearLayout>
3 ) Create thirdscrren.xml
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="vertical" >
<TextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:textColor="#898989"
android:textStyle="bold"
android:textSize="16sp"
android:text="Thanks you , you have purchased these products" />
<TextView
android:id="@+id/showCart"
android:layout_width="wrap_content"
android:layout_height="wrap_content"/>
</LinearLayout>
4) Create String.xml
<< res >> values >> string.xml >>
<?xml version="1.0" encoding="utf-8"?>
<resources>
<string name="app_name">MVC Pattern Basics</string>
<string name="menu_settings">Settings</string>
<string name="hello_world">Hello world!</string>
</resources>
5 ) Create Firstscreen.java
package com.androidexample.mvc;
import android.os.Bundle;
import android.util.Log;
import android.view.View;
import android.view.View.OnClickListener;
import android.widget.Button;
import android.widget.LinearLayout;
import android.widget.TextView;
import android.widget.Toast;
import android.app.ActionBar.LayoutParams;
import android.app.Activity;
import android.content.Intent;
public class FirstScreen extends Activity {
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.firstscreen);
final LinearLayout lm = (LinearLayout) findViewById(R.id.linearMain);
final Button secondBtn = (Button) findViewById(R.id.second);
final Controller aController = (Controller) getApplicationContext();
ModelProducts productObject = null;
for(int i=1;i<=4;i++)
{
int price = 10+i;
productObject = new ModelProducts("Product "+i,"Description "+i,price);
aController.setProducts(productObject);}
int ProductsSize = aController.getProductsArraylistSize();
LinearLayout.LayoutParams params = new LinearLayout.LayoutParams(
LayoutParams.WRAP_CONTENT, LayoutParams.WRAP_CONTENT);
for(int j=0;j< ProductsSize;j++)
{
String pName = aController.getProducts(j).getProductName();
int pPrice = aController.getProducts(j).getProductPrice();
LinearLayout ll = new LinearLayout(this);
ll.setOrientation(LinearLayout.HORIZONTAL);
TextView product = new TextView(this);
product.setText(" "+pName+" ");
ll.addView(product);
TextView price = new TextView(this);
price.setText(" $"+pPrice+" ");
ll.addView(price);
final Button btn = new Button(this);
btn.setId(j+1);
btn.setText("Add To Cart");
btn.setLayoutParams(params);
final int index = j;
btn.setOnClickListener(new OnClickListener() {
public void onClick(View v) {
Log.i("TAG", "index :" + index);
ModelProducts tempProductObject = aController.getProducts(index);
if(!aController.getCart().checkProductInCart(tempProductObject))
{
btn.setText("Added");
aController.getCart().setProducts(tempProductObject);
Toast.makeText(getApplicationContext(), "Now Cart size: "+aController.getCart().getCartSize(),
Toast.LENGTH_LONG).show();
}
else
{
Toast.makeText(getApplicationContext(), "Product "+(index+1)+" already added in cart.", Toast.LENGTH_LONG).show();
}
}
});
ll.addView(btn);
lm.addView(ll);
}
secondBtn.setOnClickListener(new OnClickListener() {
public void onClick(View v) {
Intent i = new Intent(getBaseContext(), SecondScreen.class);
startActivity(i);
}
});
}
}
6 ) Create Secondscreen.java
package com.androidexample.mvc;
import android.app.Activity;
import android.content.Intent;
import android.os.Bundle;
import android.view.View;
import android.view.View.OnClickListener;
import android.widget.Button;
import android.widget.TextView;
import android.widget.Toast;
public class SecondScreen extends Activity {
@Override
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.secondscreen);
TextView showCartContent = (TextView) findViewById(R.id.showCart);
final Button thirdBtn = (Button) findViewById(R.id.third);
final Controller aController = (Controller) getApplicationContext();
final int cartSize = aController.getCart().getCartSize();
String showString = "";
if(cartSize >0)
{
for(int i=0;i<cartSize;i++)
{
String pName = aController.getCart().getProducts(i).getProductName();
int pPrice = aController.getCart().getProducts(i).getProductPrice();
String pDisc = aController.getCart().getProducts(i).getProductDesc();
showString += "\n\nProduct Name : "+pName+"\n"+
"Price : "+pPrice+"\n"+
"Discription : "+pDisc+""+
"\n -----------------------------------";
}
}
else
showString = "\n\nShopping cart is empty.\n\n";
showCartContent.setText(showString);
thirdBtn.setOnClickListener(new OnClickListener() {
public void onClick(View v) {
if(cartSize >0)
{
Intent i = new Intent(getBaseContext(), ThirdScreen.class);
startActivity(i);
}
else
Toast.makeText(getApplicationContext(),
"Shopping cart is empty.",
Toast.LENGTH_LONG).show();
}
});
}
@Override
protected void onDestroy() {
super.onDestroy();
}
}
7 ) Create Thirdscreen.java
package com.androidexample.mvc;
import android.app.Activity;
import android.os.Bundle;
import android.widget.TextView;
public class ThirdScreen extends Activity {
@Override
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.thirdscreen);
TextView showCartContent = (TextView) findViewById(R.id.showCart);
final Controller aController = (Controller) getApplicationContext();
int cartSize = aController.getCart().getCartSize();
String showString = "";
for(int i=0;i<cartSize;i++)
{
String pName = aController.getCart().getProducts(i).getProductName();
int pPrice = aController.getCart().getProducts(i).getProductPrice();
String pDisc = aController.getCart().getProducts(i).getProductDesc();
showString += "\n\nProduct Name : "+pName+"\n"+
"Price : "+pPrice+"\n"+
"Discription : "+pDisc+""+
"\n -----------------------------------";
}
showCartContent.setText(showString);
}
}
8) Create Controller.java
package com.androidexample.mvc;
import java.util.ArrayList;
import android.app.Application;
public class Controller extends Application{
private ArrayList<ModelProducts> myProducts = new ArrayList<ModelProducts>();
private ModelCart myCart = new ModelCart();
public ModelProducts getProducts(int pPosition) {
return myProducts.get(pPosition);
}
public void setProducts(ModelProducts Products) {
myProducts.add(Products);
}
public ModelCart getCart() {
return myCart;
}
public int getProductsArraylistSize() {
return myProducts.size();
}
}
9 ) Modelcart.java
package com.androidexample.mvc;
import java.util.ArrayList;
public class ModelCart{
private ArrayList<ModelProducts> cartProducts = new ArrayList<ModelProducts>();
public ModelProducts getProducts(int pPosition) {
return cartProducts.get(pPosition);
}
public void setProducts(ModelProducts Products) {
cartProducts.add(Products);
}
public int getCartSize() {
return cartProducts.size();
}
public boolean checkProductInCart(ModelProducts aProduct) {
return cartProducts.contains(aProduct);
}
}
10 ) Create Modelproducts.java
package com.androidexample.mvc;
public class ModelProducts {
private String productName;
private String productDesc;
private int productPrice;
public ModelProducts(String productName,String productDesc,int productPrice)
{
this.productName = productName;
this.productDesc = productDesc;
this.productPrice = productPrice;
}
public String getProductName() {
return productName;
}
public String getProductDesc() {
return productDesc;
}
public int getProductPrice() {
return productPrice;
}
}
output with Screenshots:
online cart 1;
online cart 2:
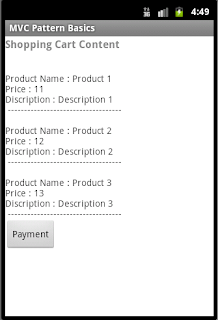
online cart 3:
Does not Work!!!
ReplyDeleteyes it is not working
ReplyDelete